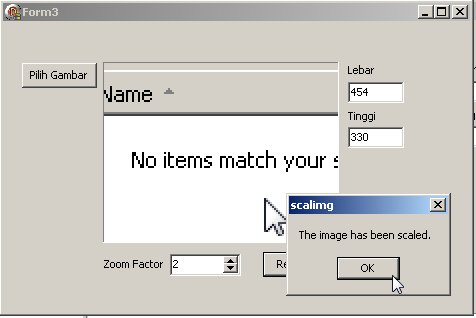
Resize Gambar (JPG & BMP) Menggunakan Delphi
Kadang kita dihadapkan dengan kasus tertentu, di mana kita harus mengubah ukuran gambar menjadi lebah besar atau lebih kecil sesuai skala yang diberikan. Nah, untuk jenis gambar JPG dan BMP, kita dapat lengsung menggunakan Windows API StretchBlt() untuk melakukan tugas ini. Berikut contoh kodenya.
Potongan kode berikut akan kita gunakan untuk mengubah ukuran gambar. Dalam contoh kita, hanya pembesaran gambar yang diberikan. Tentu saja Anda dapat membuat sendiri pengecilan gambar dengan mengubah parameter ScaleFactor menjadi bernilai antara nol dan 1.
procedure
TForm3
.
ResizeImg(Img: TImage;
const
ScaleFactor:
Integer
;
const
ImgType:
String
);
var
SrcBmp, TrgBmp: TBitmap;
W, H, NewW, NewH:
Integer
;
Jpg: TJPEGImage;
begin
//before resize the image, we have to make sure it is a Bitmap.
//If it is JPEG, we've got to convert it to Bitmap. If it is any type
//else, do not resize.
if
(ImgType<>
'.BMP'
)
and
(ImgType<>
'.JPG'
)
then
begin
raise
Exception
.
Create(
'Sorry. Image type is not supported.'
);
exit;
end
;
SrcBmp := TBitmap
.
Create;
TrgBmp := TBitmap
.
Create;
try
SrcBmp
.
Assign(Img
.
Picture
.
Graphic );
W := SrcBmp
.
Width;
H := SrcBmp
.
Height;
NewW := W * ScaleFactor;
NewH := H * ScaleFactor;
TrgBmp
.
Width := NewW;
TrgBmp
.
Height := NewH;
if
not
StretchBlt(TrgBmp
.
Canvas
.
Handle,
0
,
0
,
NewW, NewH, SrcBmp
.
Canvas
.
Handle,
0
,
0
,
W, H, SRCCOPY)
then
raise
Exception
.
Create(
'Scale failed: '
+ SysErrorMessage(GetLastError()))
else
begin
if
ImgType =
'.JPG'
then
begin
Jpg := TJPEGImage
.
Create;
try
Jpg
.
Assign(TrgBmp);
Img
.
Picture
.
Graphic
.
Assign(Jpg);
finally
Jpg
.
Free;
end
;
end
else
Img
.
Picture
.
Graphic
.
Assign(TrgBmp);
end
;
finally
SrcBmp
.
Free;
TrgBmp
.
Free;
Img
.
Refresh;
end
;
end
;
Nah, itu saja kodenya. Sebenarnya hanya perlu sekitar 5 bbaris saja, tapi karena kita harus mengkonversi antara JPG dan Bitmap, maka jadinya agak panjang.
Sekarang kita coba gunakan kode di atas:
ResizeImg(Image1, SpinEdit1
.
Value, CURRENT_IMG_TYPE);
Edit1
.
Text := IntToStr(Image1
.
Picture
.
Width) ;
Edit2
.
Text := IntToStr(Image1
.
Picture
.
Height) ;
ShowMessage(
'The image has been scaled.'
);
Di mana parameter Image1 adalah komponen TImage yang akan diubah ukuran gambarnya, SpinEdit1.Value menjadi ScaleFactor (skala) pengubahan ukuran dan CURRENT_IMG_TYPE berisi ‘.JPG’ atau ‘.BMP’ sesuai dengan file gambar yang dipilih oleh user.
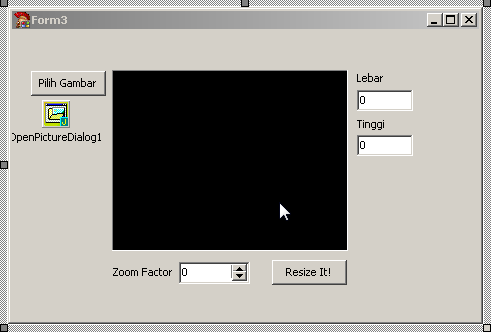
Image1 harus telah berisi gambar. Sedangkan CURRENT_IMG_TYPE harus dideklarasikan menjadi variabel global. Saya mendeklarasikannya segera setelah klause implementation.
implementation {$R *.dfm} var CURRENT_IMG_TYPE : String ; |
Berikut kode untuk memilih file gambar:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | procedure TForm3 . Button1Click(Sender: TObject); begin OpenPictureDialog1 . Filter := 'Bitmap (*.bmp)|*.bmp|JPEG Files (*.jpg)|*.jpg' ; OpenPictureDialog1 . FilterIndex := 1 ; if not OpenPictureDialog1 . Execute(Handle) then exit; CURRENT_IMG_TYPE := UpperCase(ExtractFileExt(OpenPictureDialog1 . FileName)); //ShowMessage(CURRENT_IMG_TYPE); if (CURRENT_IMG_TYPE<> '.BMP' ) and (CURRENT_IMG_TYPE<> '.JPG' ) then begin raise Exception . Create( 'Sorry. Invalid image type was selected.' ); exit; end ; Image1 . Picture . LoadFromFile(OpenPictureDialog1 . FileName); Edit1 . Text := IntToStr(Image1 . Picture . Width) ; Edit2 . Text := IntToStr(Image1 . Picture . Height) ; end ; |
Kode selengkapnya sebagai berikut:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 | unit umain; interface uses Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms, Dialogs, StdCtrls, Spin, ExtCtrls, ExtDlgs, JPEG; type TForm3 = class (TForm) SpinEdit1: TSpinEdit; Edit1: TEdit; Edit2: TEdit; Label1: TLabel; Label2: TLabel; Button1: TButton; OpenPictureDialog1: TOpenPictureDialog; Panel1: TPanel; Image1: TImage; Label3: TLabel; Button2: TButton; procedure FormCreate(Sender: TObject); procedure Button1Click(Sender: TObject); procedure Button2Click(Sender: TObject); private { Private declarations } procedure ResizeImg(Img: TImage; const ScaleFactor: Integer ; const ImgType: String ); public { Public declarations } end ; var Form3: TForm3; implementation {$R *.dfm} var CURRENT_IMG_TYPE : String ; procedure TForm3 . Button1Click(Sender: TObject); begin OpenPictureDialog1 . Filter := 'Bitmap (*.bmp)|*.bmp|JPEG Files (*.jpg)|*.jpg' ; OpenPictureDialog1 . FilterIndex := 1 ; if not OpenPictureDialog1 . Execute(Handle) then exit; CURRENT_IMG_TYPE := UpperCase(ExtractFileExt(OpenPictureDialog1 . FileName)); //ShowMessage(CURRENT_IMG_TYPE); if (CURRENT_IMG_TYPE<> '.BMP' ) and (CURRENT_IMG_TYPE<> '.JPG' ) then begin raise Exception . Create( 'Sorry. Invalid image type was selected.' ); exit; end ; Image1 . Picture . LoadFromFile(OpenPictureDialog1 . FileName); Edit1 . Text := IntToStr(Image1 . Picture . Width) ; Edit2 . Text := IntToStr(Image1 . Picture . Height) ; end ; procedure TForm3 . Button2Click(Sender: TObject); begin ResizeImg(Image1, SpinEdit1 . Value, CURRENT_IMG_TYPE); Edit1 . Text := IntToStr(Image1 . Picture . Width) ; Edit2 . Text := IntToStr(Image1 . Picture . Height) ; ShowMessage( 'The image has been scaled.' ); end ; procedure TForm3 . FormCreate(Sender: TObject); begin SpinEdit1 . MinValue := 2 ; SpinEdit1 . MaxValue := 5 ; //SpinEdit1.ReadOnly := True; SpinEdit1 . Value := 2 ; end ; procedure TForm3 . ResizeImg(Img: TImage; const ScaleFactor: Integer ; const ImgType: String ); var SrcBmp, TrgBmp: TBitmap; W, H, NewW, NewH: Integer ; Jpg: TJPEGImage; begin //before resize the image, we have to make sure it is a Bitmap. //If it is JPEG, we've got to convert it to Bitmap. If it is any type //else, do not resize. if (ImgType<> '.BMP' ) and (ImgType<> '.JPG' ) then begin raise Exception . Create( 'Sorry. Image type is not supported.' ); exit; end ; SrcBmp := TBitmap . Create; TrgBmp := TBitmap . Create; try SrcBmp . Assign(Img . Picture . Graphic ); W := SrcBmp . Width; H := SrcBmp . Height; NewW := W * ScaleFactor; NewH := H * ScaleFactor; TrgBmp . Width := NewW; TrgBmp . Height := NewH; if not StretchBlt(TrgBmp . Canvas . Handle, 0 , 0 , NewW, NewH, SrcBmp . Canvas . Handle, 0 , 0 , W, H, SRCCOPY) then raise Exception . Create( 'Scale failed: ' + SysErrorMessage(GetLastError())) else begin if ImgType = '.JPG' then begin Jpg := TJPEGImage . Create; try Jpg . Assign(TrgBmp); Img . Picture . Graphic . Assign(Jpg); finally Jpg . Free; end ; end else Img . Picture . Graphic . Assign(TrgBmp); end ; finally SrcBmp . Free; TrgBmp . Free; Img . Refresh; end ; end ; end . |
Bila dijalankan, kita akan mendapatkan sebuah aplikasi sederhana untuk mengubah ukuran gambar sesuai skala tertentu.
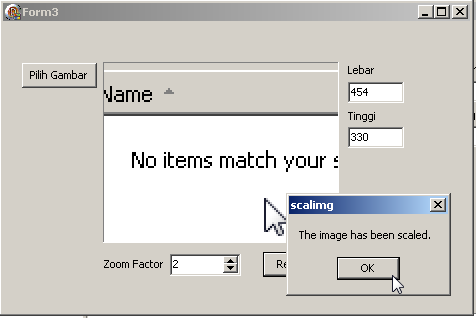
Comments